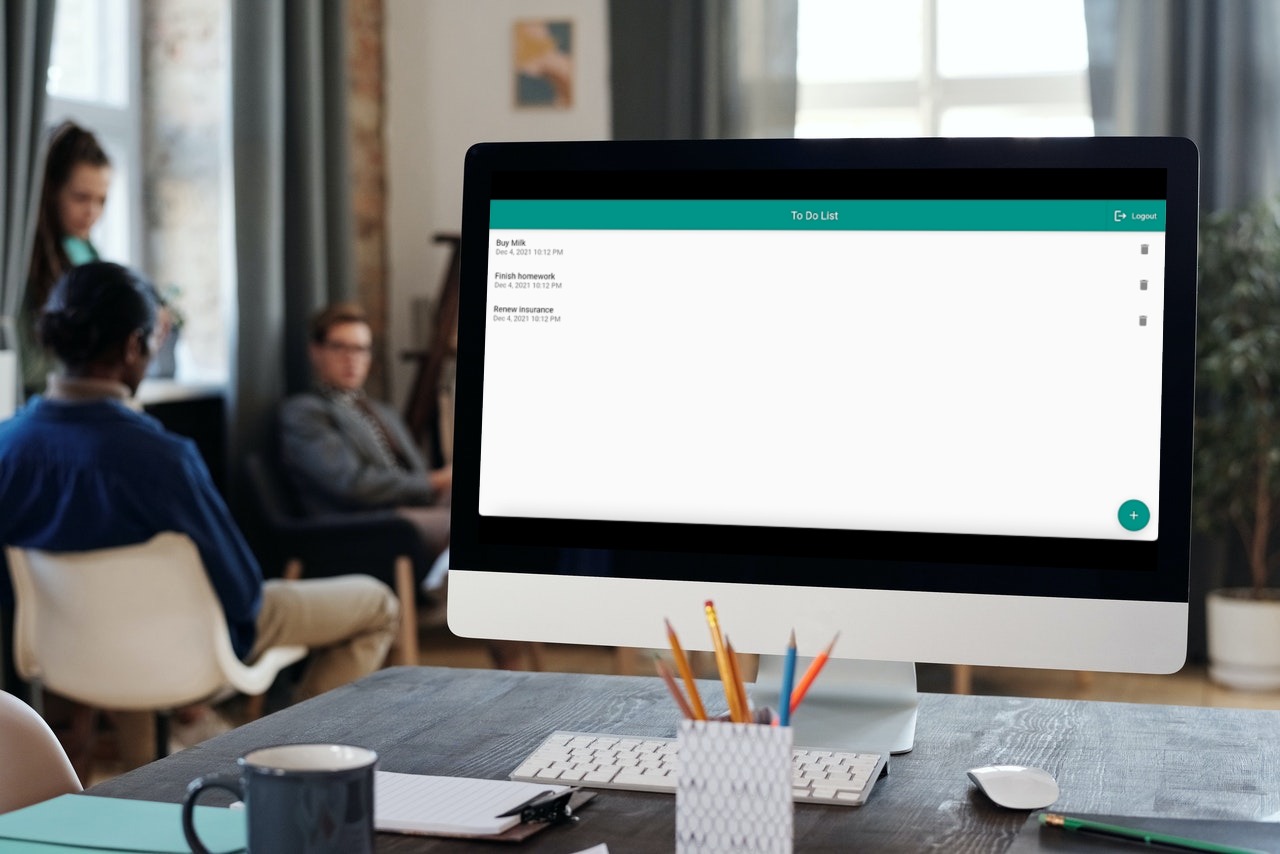
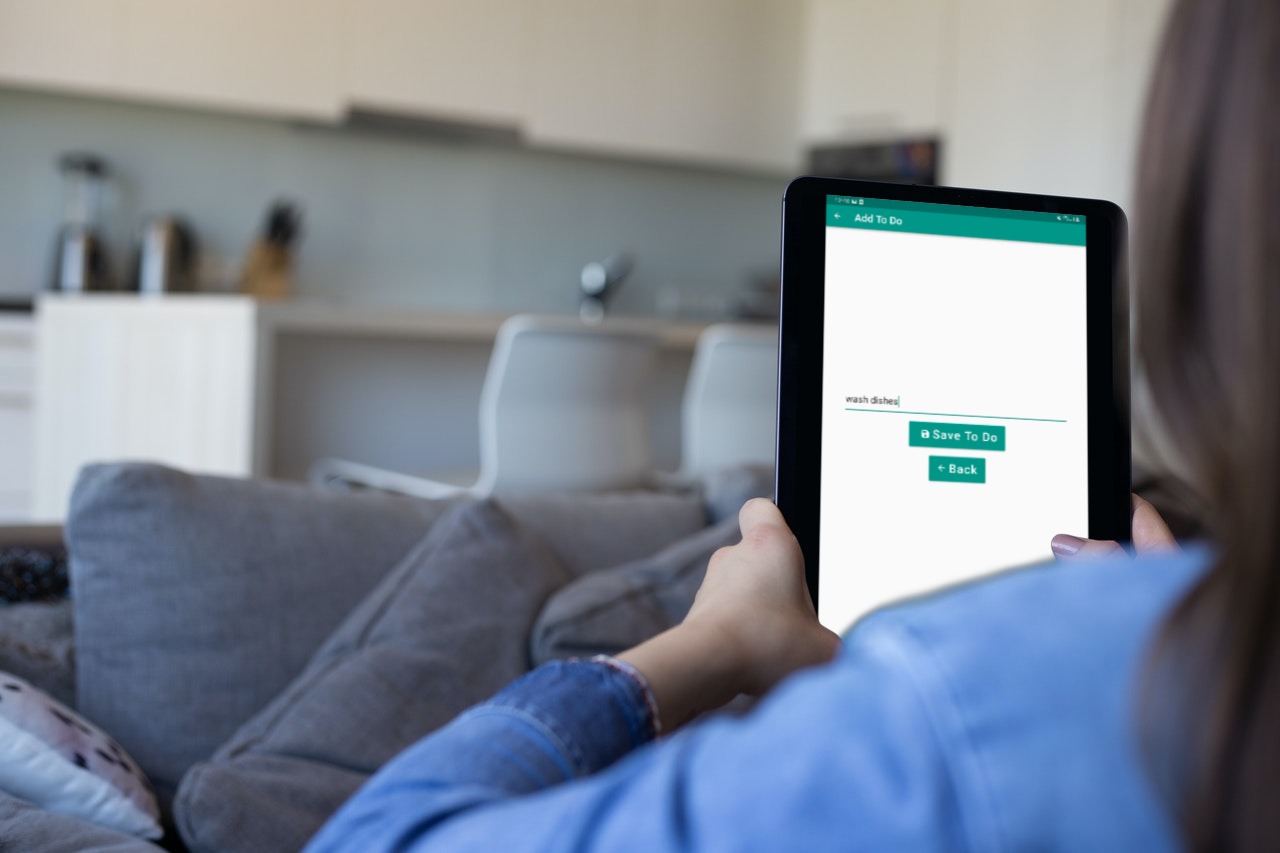
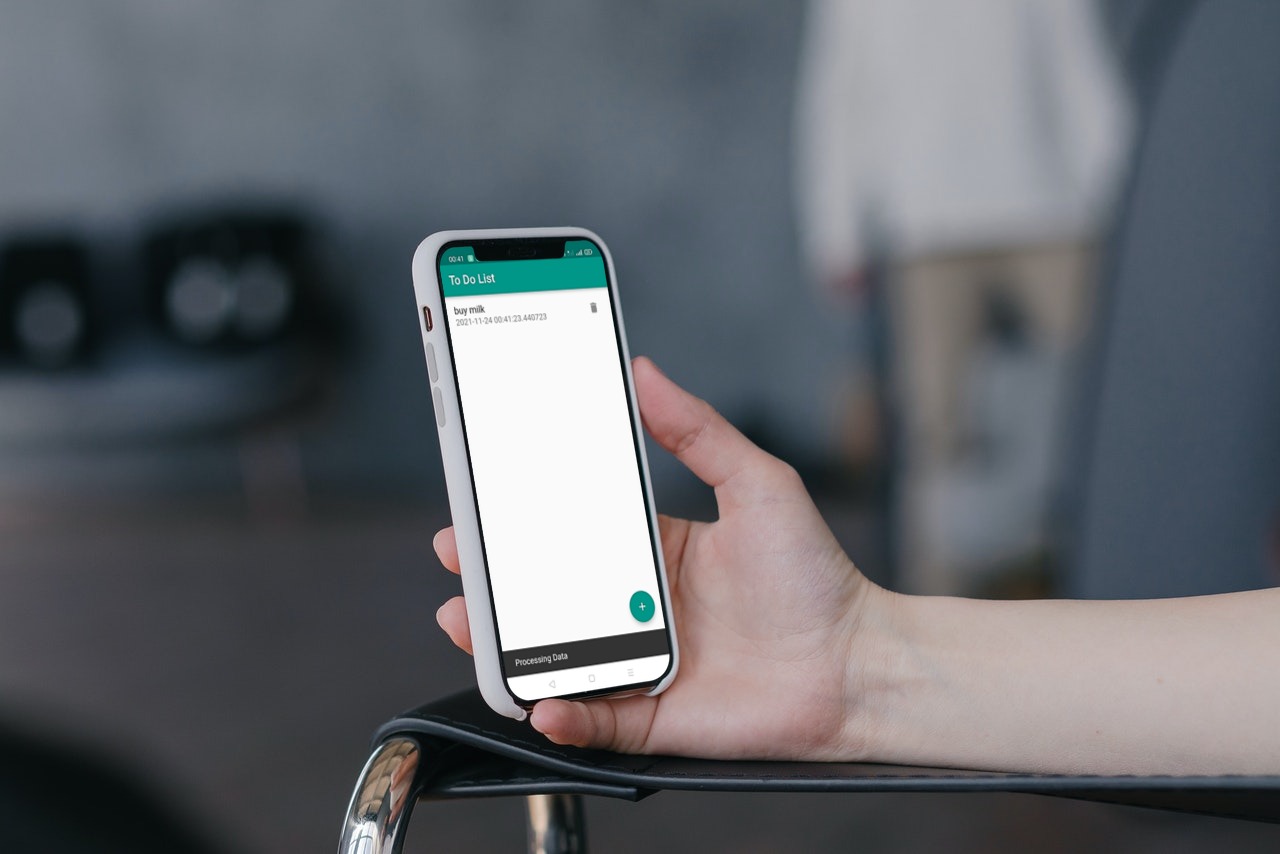
Project links
- Github (and Documentation): https://github.com/MichaelRinglein/ToDoList
- Live Demo: https://flutterwebapps.com/portfolio/to-do-list/#/
- Play Store: https://play.google.com/store/apps/details?id=com.strawanzer.to_do_list
To Do List | Progressive Web App, Android, Firebase Firestore, Firebase Authentication
A user can create to do's to a list, save them and delete them.
Packages used
- Firebase Auth
- Firebase Google Sign In
- Firebase Firestore
- Provider
Documentation
main.dart
Firebase is initialized here on the top of the widget tree. A Futurebuilder is used, the future is the Firebase.initializeApp()
future. On successful connection to Firebase the next Widget in the tree is shown.
home.dart
This Widget is between the main MyApp() and all the following widgets and pages. A StreamBuilder is listening to theauthStateChanges()
stream of Firebase. If the user is logged in, the Home() widget is shown. If he is logged out / hasn't registered yet, the SignIn() widget is shown.
loading.dart
A Flutter CircularProgressIndicator
is shown with a Text
widget below. The Loading()
class is a stateless widget with the loadingText
as parameter. The Loading
widget can be inserted everywhere in the app with a customnized loadingText
depending on the task where this widget is inserted.
auth/sign_in.dart
The screen to either sign in anonymously or with Google. UniversalPlatform.isWeb
and UniversalPlatform.isAndroid
are used to iscriminate between web and native.
database/auth.dart
The Firebase authentication methods are stored here. I prefer Future classes with try-catch loops so the app doesn’t crash and the user gets an error (from the sign_in.dart) in case the authentication doesn’t work.
database/database.dart
In this file are all Firebase related queries.
The addToDoItem
takes in the toDo
and the user
as parameters and adds the to do via the .set
method from Firebase.
The editToDo
takes in the toDo
and the user
as parameters and updates a to do via the .update
method from Firebase.
The deleteToDo
takes in the toDo
and the user
as parameters and deletes a to do via the .delete method from Firebase.
pages/add_to_do.dart
A stateful Widget is used. The Widget gets the User
via Provider
. Inside this is a Form
with a single field (the to do which should be added).
services/get_to_do_list.dart
A stateful Widget is used. The Widget gets the User
via Provider
. The to do's are provided by a StreamBuilder
and displayed via a ListView
. The Stream for the StreamProvider is taken straight from FirebaseFirestore
.